Code coverage is a way to measure how much of your Java code is tested. If you have 80% code coverage, it means 80% of your code has been executed by tests at least once. But what does that really mean?
Understanding Code Coverage
Think of your code as a giant mansion with 100 rooms. Code coverage tells you how many rooms have been visited. If your tests have entered 80 rooms, that’s 80% coverage! But just stepping inside doesn’t mean you’ve explored every corner.
Code coverage is usually measured using tools like:
- JaCoCo
- Cobertura
- Emma
These tools analyze your tests and tell you how much of your code has actually been run.
What 80% Coverage Tells You
Having 80% coverage means most of your code was executed by tests. But it doesn’t guarantee perfect quality. Let’s break it down with an example.
public int add(int a, int b) { if (a > 0 && b > 0) { return a + b; } else { return 0; } }
If your test only checks add(2,3)
, the if
condition is covered. But what about when a
or b
is negative? That part may not be tested!
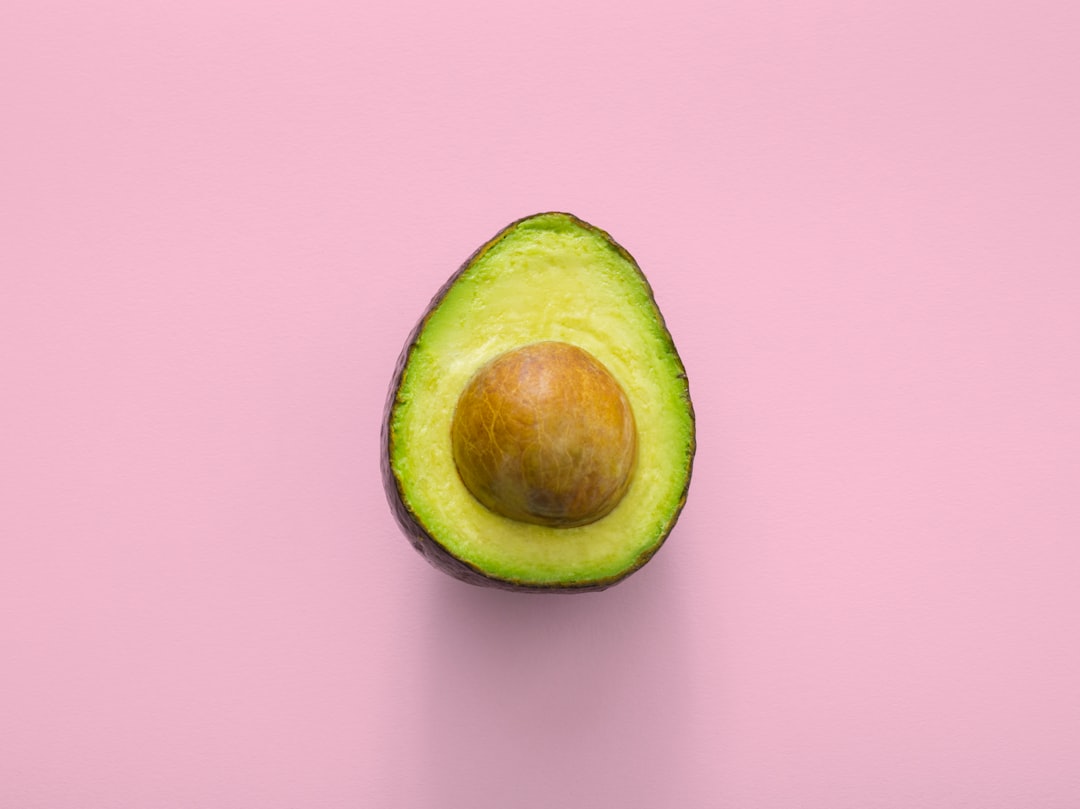
Different Types of Code Coverage
Not all coverage is the same. There are various types:
- Line Coverage: Measures how many lines of code are executed.
- Branch Coverage: Checks if both true and false paths of conditions are tested.
- Path Coverage: Examines all possible execution paths (hard to achieve fully).
So, if you have 80% line coverage, it doesn’t mean every branch has been tested.
80% – Good or Bad?
It depends! Generally, 80% is considered a healthy target. But focusing only on the percentage can be misleading.
What really matters is:
- Are critical parts of the code tested?
- Are edge cases covered?
- Are error scenarios tested?
Sometimes, you can have high coverage but poor tests!
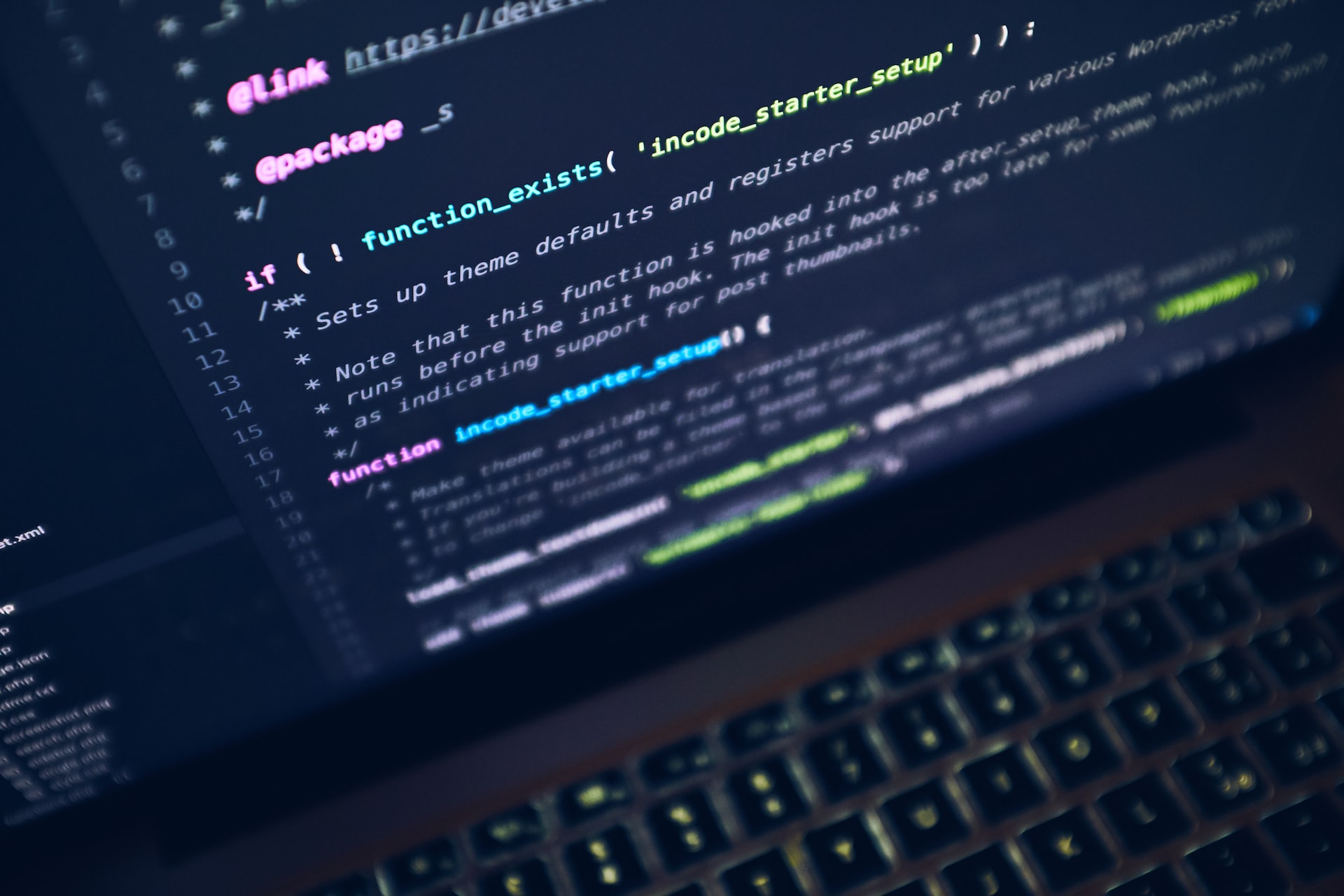
Should You Aim for 100%?
Reaching 100% can be impractical. Some parts of the code, like logging or error handling, are tough to test.
Instead of chasing a perfect number, focus on writing effective tests. Quality over quantity!
How to Improve Test Coverage
Want better test coverage? Here are some tips:
- Write tests when you write code, not after.
- Use tools like JaCoCo to check coverage regularly.
- Test different scenarios, not just happy paths.
- Don’t just aim for percentage—test for logic!
Conclusion
80% code coverage means most of your code is executed during tests. But it’s not a silver bullet. Focus on meaningful tests, not just numbers!
Happy coding!