Creating a pixel distortion effect in Three.js can add a dynamic and abstract appearance to your 3D scenes. This effect is widely used in digital art, web animations, and interactive experiences. Three.js, a powerful JavaScript library for 3D rendering, allows developers to manipulate shaders to achieve this visual effect.
Understanding the Pixel Distortion Effect
The pixel distortion effect works by displacing pixels in a fragment shader. By modifying the pixel positions based on noise, time, or texture coordinates, we can simulate glitches, wave distortions, or digital interference. The key components of this effect include:
- Vertex and Fragment Shaders: These shaders help manipulate pixel positions and colors.
- Noise Functions: Used to create irregular movement and distortion.
- Time-Based Animation: Applying time-based offsets to achieve a dynamic effect.
To achieve this effect efficiently, Three.js provides tools such as the ShaderMaterial class, which allows custom shaders to be integrated into a scene.
Setting Up Your Three.js Scene
The first step to implementing a pixel distortion effect is setting up a basic Three.js scene. Start by including the Three.js library in your HTML file:
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
Then, initialize the scene, camera, and renderer:
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
camera.position.z = 5;
Creating the Pixel Distortion Shader
We need a custom shader to manipulate the pixels using GLSL (OpenGL Shading Language). Below is an example of a fragment shader that applies a distortion effect:
const vertexShader = `
varying vec2 vUv;
void main() {
vUv = uv;
gl_Position = projectionMatrix * modelViewMatrix * vec4(position, 1.0);
}
`;
const fragmentShader = `
varying vec2 vUv;
uniform float time;
void main() {
vec2 distortedUv = vUv;
distortedUv.x += sin(distortedUv.y * 10.0 + time) * 0.02;
gl_FragColor = vec4(distortedUv, 1.0, 1.0);
}
`;
Here, the time
uniform is used to animate the distortion over time.
Applying the Shader to a Material
We now apply the custom shader to a plane that will serve as the display surface:
const shaderMaterial = new THREE.ShaderMaterial({
vertexShader,
fragmentShader,
uniforms: {
time: { value: 0.0 }
}
});
const geometry = new THREE.PlaneGeometry(2, 2);
const mesh = new THREE.Mesh(geometry, shaderMaterial);
scene.add(mesh);
To animate the effect, we update the time uniform in the render loop:
function animate() {
requestAnimationFrame(animate);
shaderMaterial.uniforms.time.value += 0.05;
renderer.render(scene, camera);
}
animate();
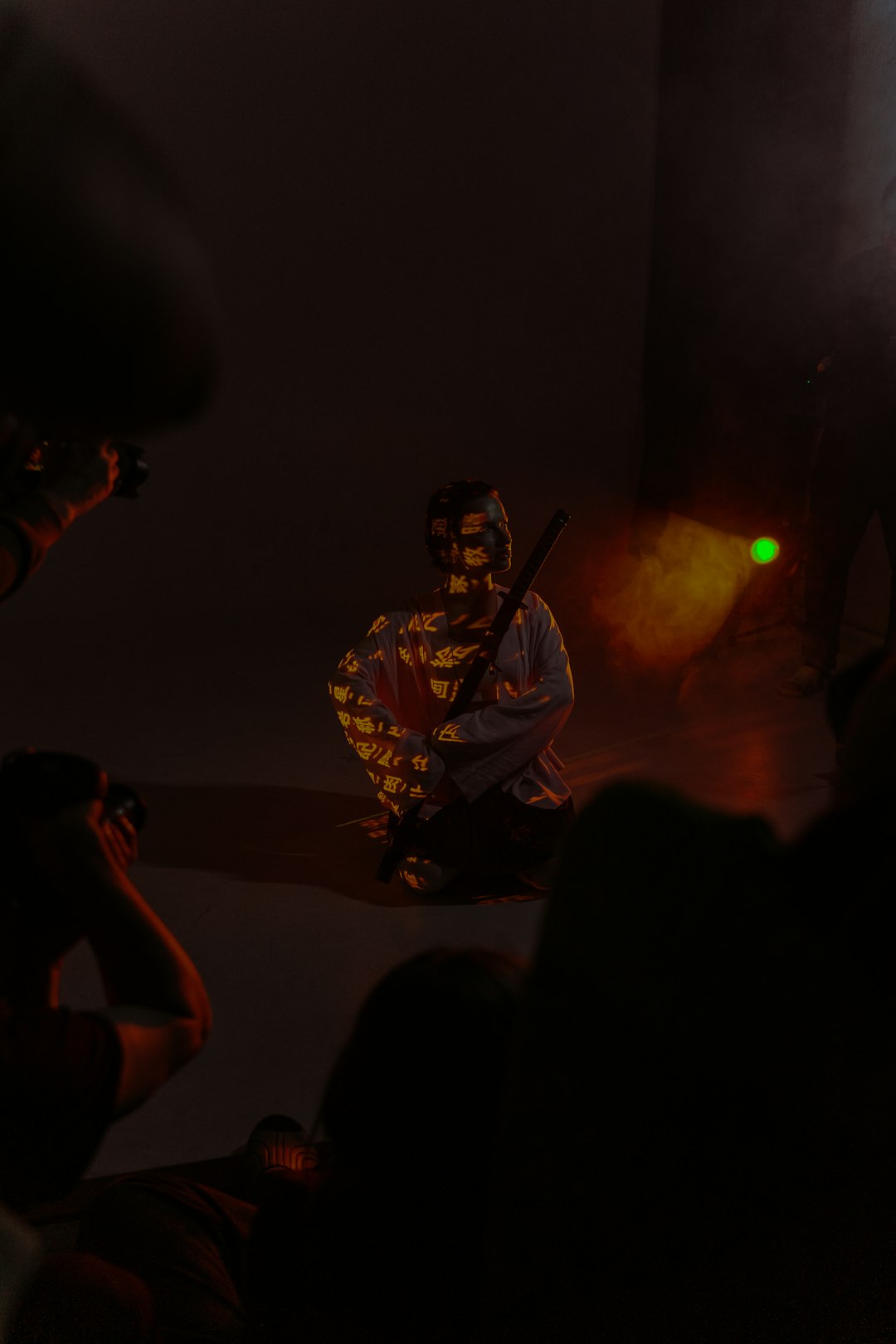
Enhancing the Effect with Noise
Adding noise enhances the distortion and creates more organic movement. We can integrate Perlin or Simplex noise to achieve a glitch-like effect. Modify the fragment shader as follows:
uniform float time;
float random(vec2 p) {
return fract(sin(dot(p, vec2(12.9898, 78.233))) * 43758.5453);
}
void main() {
vec2 distortedUv = vUv;
float noise = random(distortedUv + time);
distortedUv.x += sin(distortedUv.y * 10.0 + time) * 0.02 + noise * 0.02;
gl_FragColor = vec4(distortedUv, 1.0, 1.0);
}
The random
function generates pseudo-random values, which, when combined with sine waves, create an aesthetically pleasing digital distortion.
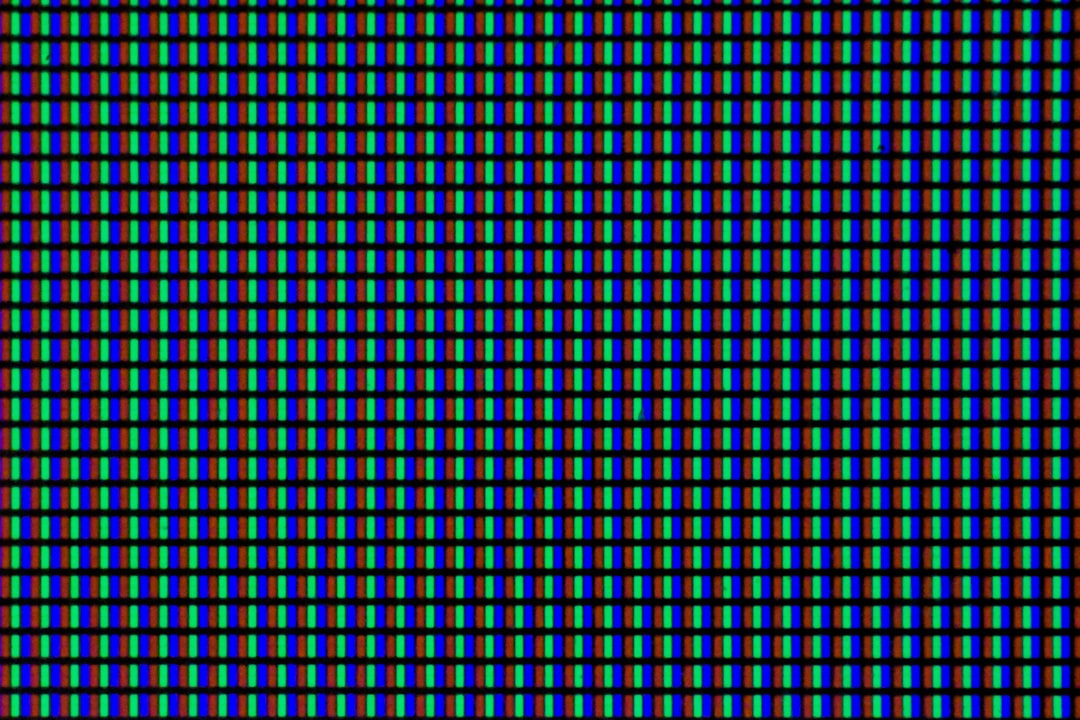
Final Adjustments and Optimization
Before deploying the effect, consider optimizing performance:
- Use WebGLRenderer settings to reduce unnecessary calculations.
- Optimize shader execution by minimizing expensive operations.
- Consider modularizing shader code for better reusability.
By tweaking the distortion parameters, shader complexity, and texture inputs, you can create unique variations suitable for your application.
Conclusion
The pixel distortion effect in Three.js opens up various creative possibilities in digital design. By understanding the fundamentals of shaders, noise functions, and time-based animation, you can craft compelling visuals that enhance your interactive projects.