In recent years, AI-powered chatbots have become an essential tool for businesses looking to enhance customer service, automate responses, and streamline communication. By integrating OpenAI’s ChatGPT with PHP, you can create a highly functional chatbot capable of understanding and interacting with users in a natural way.
Why Use AI for a Chatbot?
Traditional rule-based chatbots often struggle to provide meaningful conversations due to their limited responses and rigid structures. With AI-powered models like ChatGPT, chatbots can:
- Understand natural language and context.
- Offer dynamic and engaging conversations.
- Provide personalized responses based on user interactions.
- Improve customer support by offering 24/7 availability.
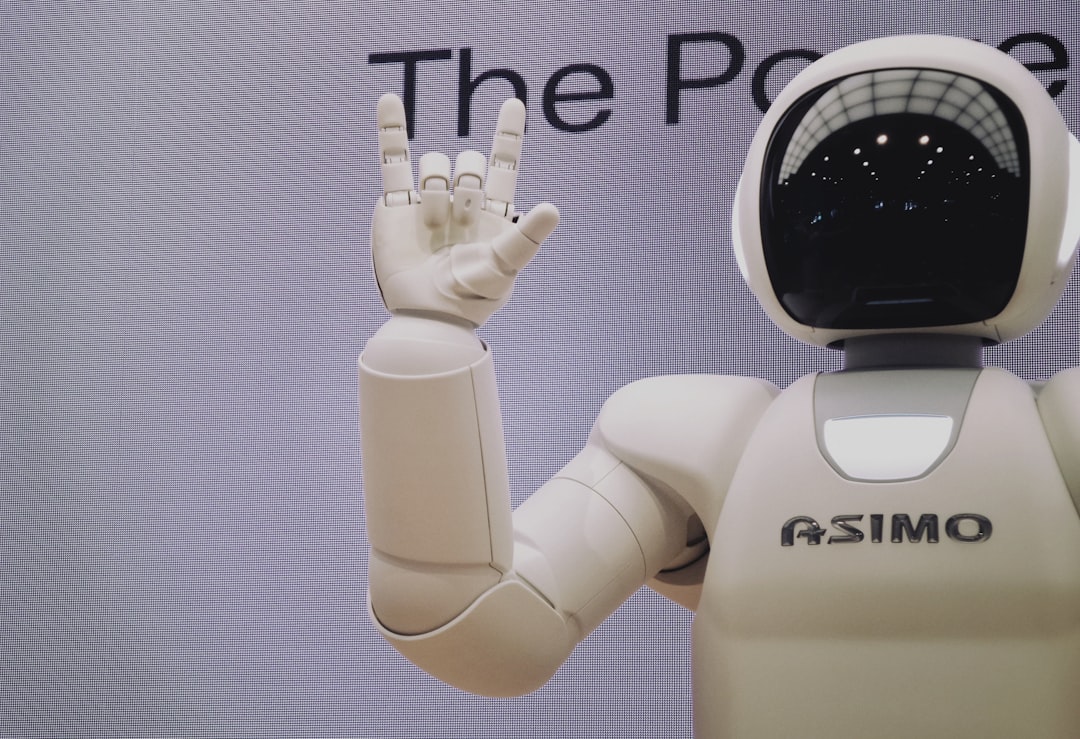
Setting Up Your AI Chatbot with ChatGPT and PHP
To build a chatbot using AI and PHP, you’ll need access to OpenAI’s API and some basic setup to handle requests and responses efficiently.
Step 1: Obtain API Access
To interact with ChatGPT, you need an API key from OpenAI. If you don’t already have an API key, follow these steps:
- Go to OpenAI’s website and sign up.
- Once logged in, navigate to the API section.
- Generate a new API key and store it securely.
Step 2: Create the PHP Script
Now, let’s set up a PHP script to send user input to the ChatGPT API and receive a response. Below is a basic example:
<?php
$apiKey = 'your_api_key_here';
$inputText = 'Hello, how can I help you?';
$data = [
'model' => 'gpt-4',
'messages' => [['role' => 'user', 'content' => $inputText]],
];
$ch = curl_init('https://api.openai.com/v1/chat/completions');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Authorization: Bearer ' . $apiKey,
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
$response = curl_exec($ch);
curl_close($ch);
$responseData = json_decode($response, true);
echo $responseData['choices'][0]['message']['content'];
?>
This script sends a prompt to the API and retrieves a response, which can then be displayed to users in a chatbot interface.
Step 3: Build a Simple Frontend
To interact with the chatbot through a web interface, create a simple frontend using HTML and JavaScript.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>AI Chatbot</title>
</head>
<body>
<h2>AI Chatbot</h2>
<div id="chatbox"></div>
<input type="text" id="userInput">
<button onclick="sendMessage()">Send</button>
<script>
function sendMessage() {
let input = document.getElementById('userInput').value;
fetch('chatbot.php', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ message: input }),
})
.then(response => response.text())
.then(data => {
document.getElementById('chatbox').innerHTML += '<p>User: ' + input + '</p>';
document.getElementById('chatbox').innerHTML += '<p>Bot: ' + data + '</p>';
});
}
</script>
</body>
</html>
This simple frontend allows users to enter text, which is then sent to your PHP script for processing.
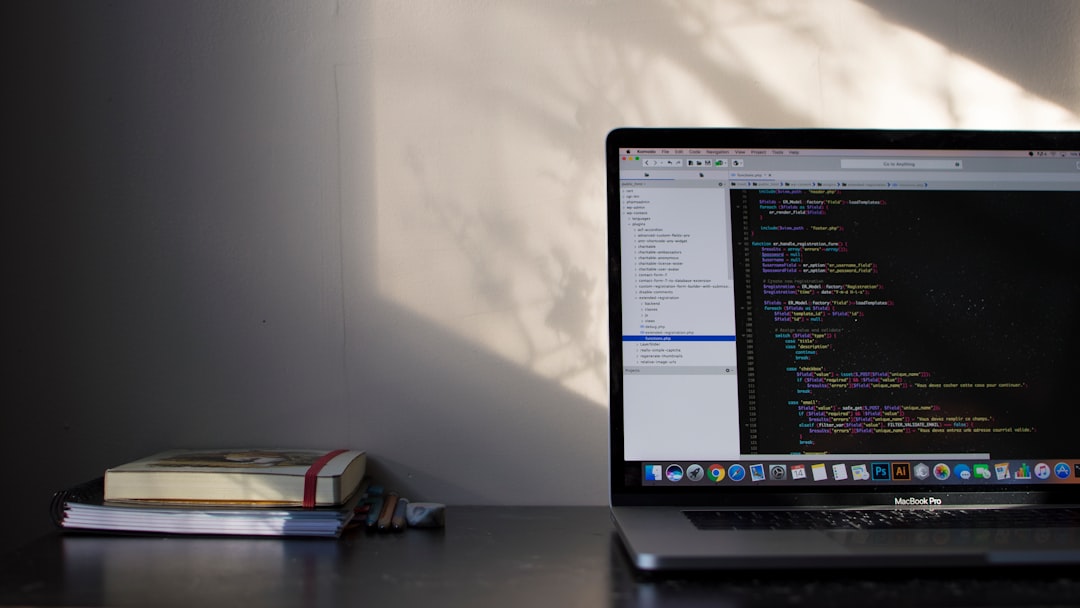
Enhancing Your Chatbot
To improve your chatbot’s functionality, consider implementing the following enhancements:
- Context Awareness: Store conversation history to provide more relevant responses.
- Database Integration: Use a database to track user interactions and provide personalized responses.
- Voice Input: Convert speech to text to enable voice-based communication.
- Integration with Other Platforms: Connect your chatbot with messaging apps like WhatsApp or Facebook Messenger.
Conclusion
Building an AI-powered chatbot using ChatGPT and PHP is a highly effective way to automate customer interactions and enhance user experience. By leveraging the power of natural language processing, your chatbot can provide meaningful, conversational responses that improve engagement and efficiency.
With further development, integrating additional features like database support, API extensions, and machine learning improvements can make your chatbot even more interactive and useful. Start experimenting with AI and PHP today to create your own intelligent chatbot solution!