Environment variables play a crucial role in managing configuration settings for applications. They help keep sensitive information like API keys, database URLs, and secret tokens outside the source code, making them an essential tool for developers. In Python, setting environment variables is straightforward, and understanding how to work with them can significantly improve the security and maintainability of your code.
What Are Environment Variables?
Environment variables are key-value pairs used to store configuration settings on a system-wide or per-process basis. These variables can be accessed by applications and scripts to retrieve essential information dynamically.
Why Use Environment Variables in Python?
Python applications often require external configurations such as:
- Database connection strings
- API keys and authentication tokens
- File paths and directory structures
- System settings based on deployment environments
Storing such information in environment variables helps keep the code clean and secure.
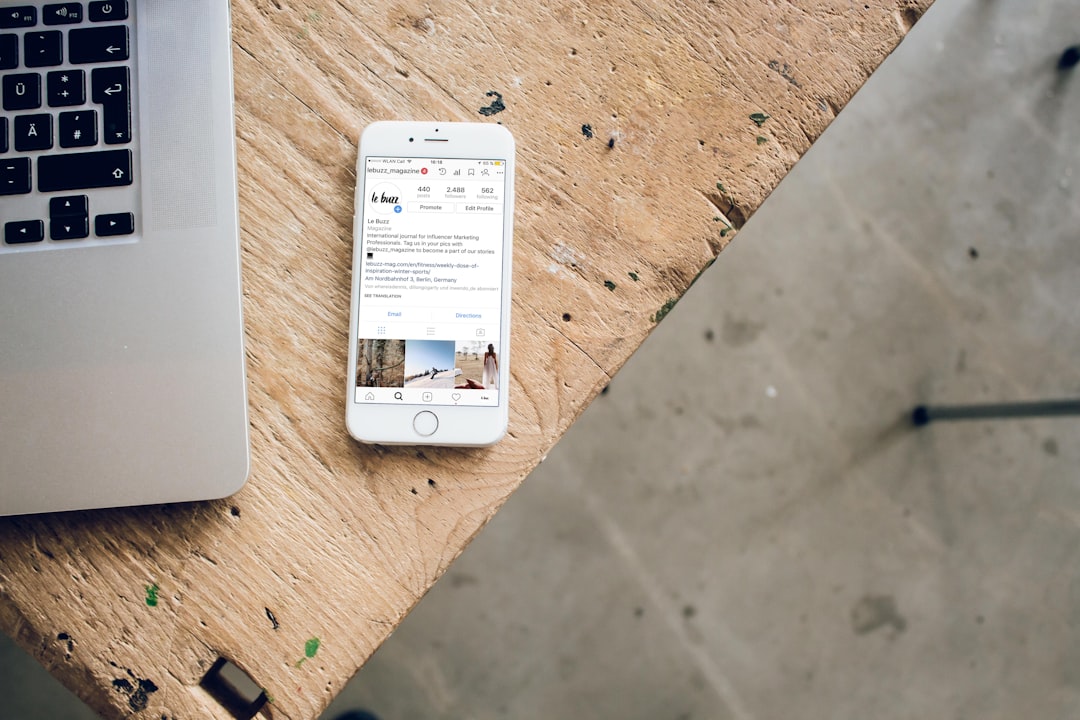
Setting Environment Variables in Python
There are multiple ways to set environment variables in Python. Let’s explore some of the most common methods.
1. Using os.environ
You can set an environment variable in Python using the os module:
import os
os.environ["MY_VARIABLE"] = "Hello, World!"
This assigns the value “Hello, World!” to the variable MY_VARIABLE. However, this change is only effective for the duration of the script execution.
2. Using os.putenv()
Another way to set an environment variable is with os.putenv():
import os
os.putenv("MY_VARIABLE", "Hello, World!")
However, os.putenv() does not update os.environ and is, therefore, less commonly used.
3. Setting Environment Variables in the Command Line
For a more persistent approach, you can set environment variables before running a Python script:
On Windows:
set MY_VARIABLE=Hello, World!
python my_script.py
On macOS/Linux:
export MY_VARIABLE="Hello, World!"
python my_script.py
This ensures the variable is available to the script during execution.
4. Using a .env File
For larger projects, storing environment variables in a .env file using the python-dotenv package is a best practice.
Install python-dotenv using pip:
pip install python-dotenv
Create a .env file:
MY_VARIABLE=Hello, World!
Load variables from the file in Python:
from dotenv import load_dotenv
import os
load_dotenv()
my_variable = os.getenv("MY_VARIABLE")
print(my_variable)
This method is especially useful for projects using version control, as you can exclude the .env file from the repository.
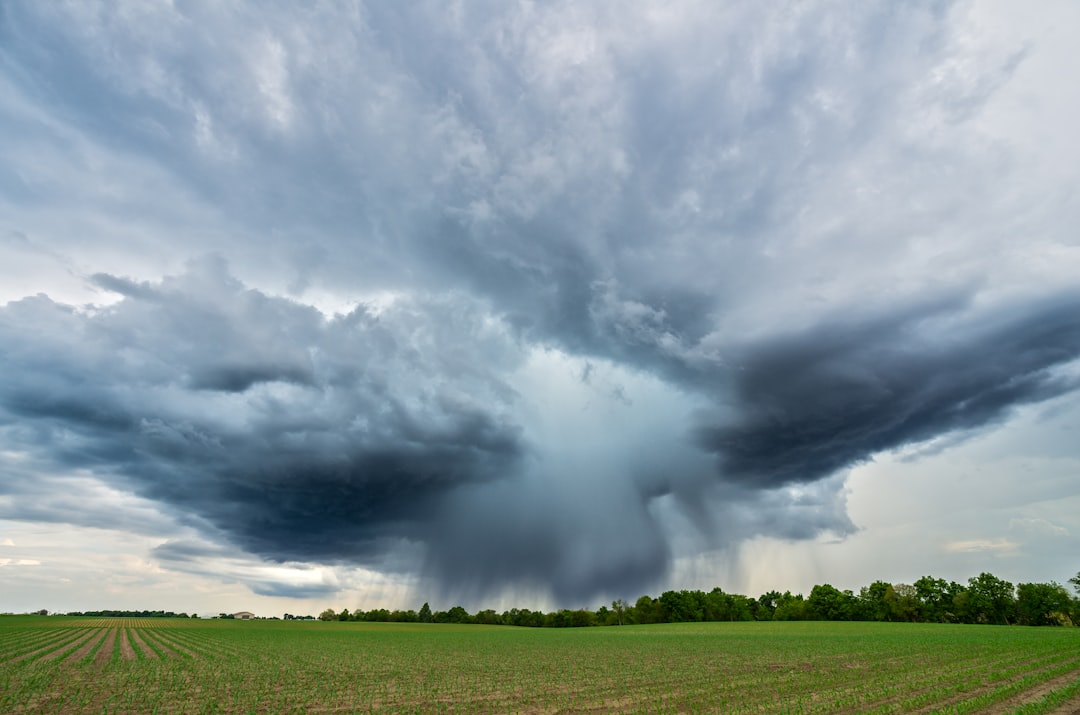
Retrieving Environment Variables
After setting an environment variable, you can retrieve it in Python using os.getenv():
import os
value = os.getenv("MY_VARIABLE")
print(value) # Output: Hello, World!
If the environment variable is not set, os.getenv() returns None, or you can provide a default value:
value = os.getenv("MY_VARIABLE", "Default Value")
Best Practices
- Keep sensitive data out of source code – Use environment variables instead of hardcoding API keys and secrets.
- Use a .env file for local development – Manage environment variables efficiently without modifying the system environment.
- Use default values – Ensure your application runs even if an environment variable is missing.
- Do not commit sensitive files – Add .env files to .gitignore to avoid exposing sensitive data in repositories.
Conclusion
Understanding how to set and retrieve environment variables in Python is an essential skill for building secure and scalable applications. Whether using os.environ, command-line exports, or a .env file, the right approach depends on your project’s needs. By following best practices, you can keep your applications configurable, secure, and easy to manage.